![[안드로이드/Android] Fragment 프래그먼트, FrameLayout 프레임 레이아웃 예제(화면 일부만 전환하기), Fragment 프](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FTi0q6%2Fbtq1pATnCqQ%2FjTXYy2jstelKT67QJD62G0%2Fimg.png)
안녕하세요!
하나의 프로젝트를 진행하다 보면 같은 액티비티가 여러 번 사용되는 경우가 생깁니다.
같은 액티비티가 아니더라도 컴포넌트 구성 요소가 비슷하거나 같아서 구성 요소가 많아질수록
코드가 복잡해지고 길어지고 화면 일부만 전환하고 싶기도 합니다.
이렇게 길고 복잡해진 코드에서 하나를 수정하기 위해 전체 중복된 모든 코드를 바꿔야하는 번거로움이 생깁니다.
그럴 때 중복된 코드를 하나의 틀로 관리해주는 것이 Fragment와 FrameLayout입니다.
오늘은 Fragment와 FrameLayout을 사용하는 실습을 진행하겠습니다.
Fragment란?
안드로이드에는 Activity와 Fragment라는 개념이 있습니다. Fragment는 하나의 Activity가 여러 개의 화면을 보여줄 수 있도록 해주는 개념인데 동작 또는 사용자 인터페이스의 일부입니다. 여러 개의 프래그먼트로 하나의 액티비티와 조합하여 사용자 인터페이스를 만들 수 있으며, 프래그먼트는 다른 액티비티에서 역시 재사용이 용이합니다.
Fragment는 activity와 다른 life-cycle을 가지고 있으며, 자체 생명 주기와 자체 입력 이벤트가 있습니다.
(다른 activity에서도 사용 가능한 "하위" activity 개념입니다)
간단한 프래그먼트 사용 예시입니다.
(다른 프레임워크의 예로 템플릿 확장의 개념입니다)
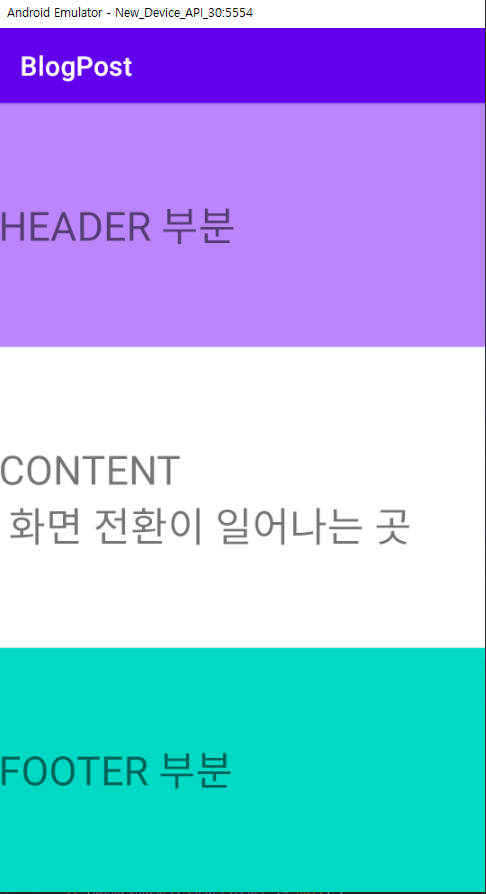
위의 activity에서 content 부분의 내용을 봐주세요.
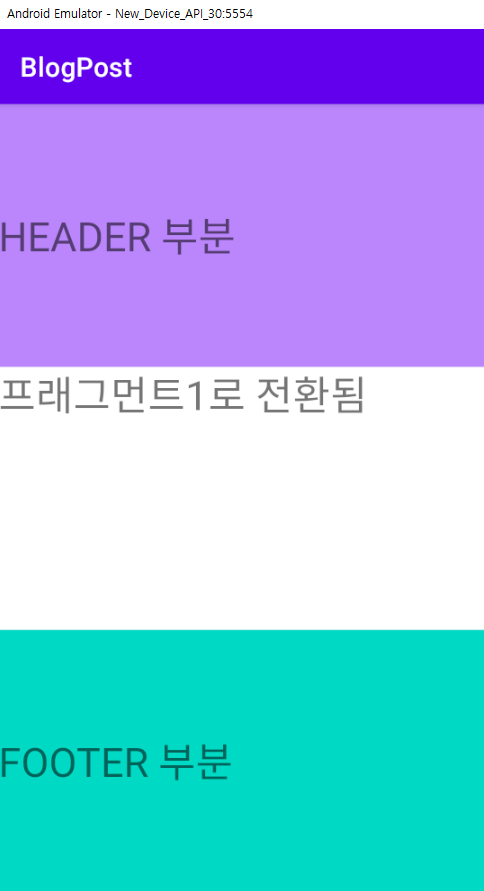
위의 activity에서 content 부분이 fragment1로 전환된 것을 확인할 수 있습니다.
Button을 이용해서 fragment1과 2로 화면 전환이 가능합니다.
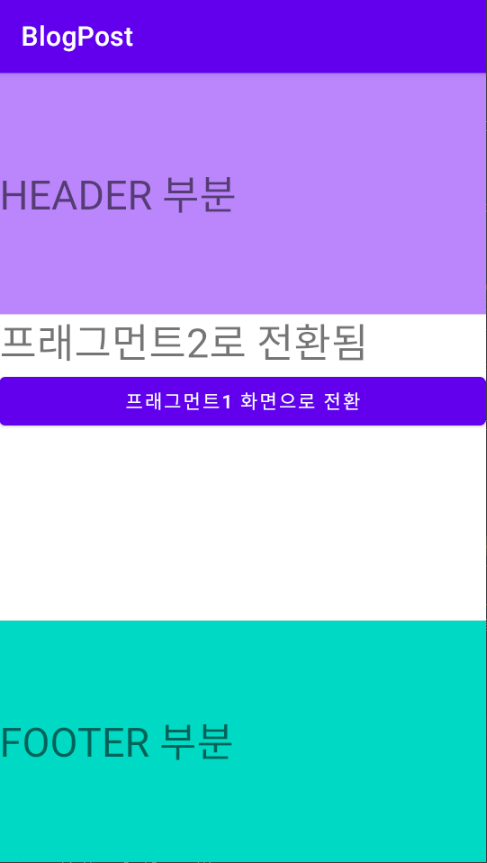
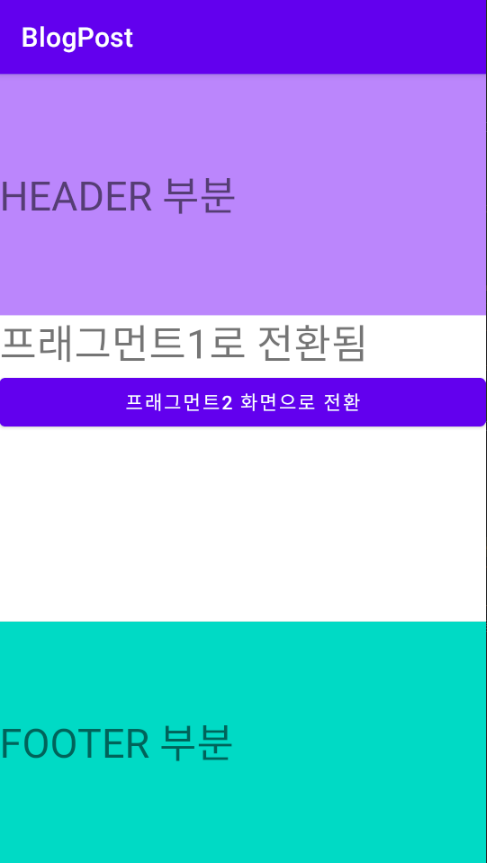
다음과 같이 버튼을 이용해서 content 부분의 fragment1과 2로 전환시킬 수 있습니다.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="1" android:background="@color/purple_200"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="30dp" android:layout_gravity="center_vertical" android:text="HEADER 부분"/> </LinearLayout> <FrameLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="1" android:id="@+id/frameLayout"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="30dp" android:layout_gravity="center_vertical" android:text=""/> </FrameLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="1" android:background="@color/teal_200"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="30dp" android:layout_gravity="center_vertical" android:text="FOOTER 부분"/> </LinearLayout> </LinearLayout>
MainActivity.java
package com.example.blogpost; import androidx.appcompat.app.AppCompatActivity; import androidx.fragment.app.FragmentTransaction; import android.os.Bundle; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); FragmentTransaction transaction = getSupportFragmentManager().beginTransaction(); // 프래그먼트매니저를 통해 사용 Fragment1 fragment1= new Fragment1(); // 객체 생성 transaction.replace(R.id.frameLayout, fragment1); //layout, 교체될 layout transaction.commit(); //commit으로 저장 하지 않으면 화면 전환이 되지 않음 } }
fragment1.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:text="프래그먼트1로 전환됨" android:textSize="30dp"/> <Button android:layout_width="match_parent" android:layout_height="wrap_content" android:text="프래그먼트2 화면으로 전환" android:id="@+id/btn_frag2"/> </LinearLayout>
fragment2.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:text="프래그먼트2로 전환됨" android:textSize="30dp"/> <Button android:layout_width="match_parent" android:layout_height="wrap_content" android:text="프래그먼트1 화면으로 전환" android:id="@+id/btn_frag1"/> </LinearLayout>
Fragment1.java
package com.example.blogpost; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.Button; import androidx.annotation.NonNull; import androidx.annotation.Nullable; import androidx.fragment.app.Fragment; import androidx.fragment.app.FragmentTransaction; public class Fragment1 extends Fragment { private View view; private Button btn_frag2; @Nullable @Override public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { view = inflater.inflate(R.layout.fragment1, container, false); btn_frag2 = view.findViewById(R.id.btn_frag2); //fragment에서는 그냥 findViewById로 Button id를 가져올 수 없음. //인플레이터된 view를 사용하여 가져옴. btn_frag2.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { FragmentTransaction transaction = getActivity().getSupportFragmentManager().beginTransaction(); Fragment2 fragment2 = new Fragment2(); transaction.replace(R.id.frameLayout, fragment2); transaction.commit(); } }); return view; } }
Fragment2.java
package com.example.blogpost; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.Button; import androidx.annotation.NonNull; import androidx.annotation.Nullable; import androidx.fragment.app.Fragment; import androidx.fragment.app.FragmentTransaction; public class Fragment2 extends Fragment { private View view; private Button btn_frag1; @Nullable @Override public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { view = inflater.inflate(R.layout.fragment2, container, false); btn_frag1 = view.findViewById(R.id.btn_frag1); btn_frag1.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { FragmentTransaction transaction = getActivity().getSupportFragmentManager().beginTransaction(); Fragment1 fragment1 = new Fragment1(); transaction.replace(R.id.frameLayout, fragment1); transaction.commit(); } }); return view; } }
이렇게 액티비티와 프래그먼트를 작성해주시면 됩니다.
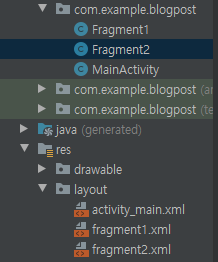
파일 구조는 이런 식으로 구성되면 됩니다.
프래그먼트의 내용이 transaction.replace로 인해 FrameLayout 부분이 교체되어 하나의 액티비티에
여러 개의 프래그먼트를 사용할 수 있게 됩니다.
오늘은 Fragment 프래그먼트, FrameLayout 프레임 레이아웃 예제(화면 일부만 전환하기), Fragment 프래그먼트 간 화면 전환, Fragment 프래그먼트에서 OnClick 사용 등의 실습을 해봤습니다.
고생하셨습니다!
'Framework > Android' 카테고리의 다른 글
[안드로이드/Android] 스피너(Spinner) 값을 이용한 레이아웃 예제 (5) | 2021.05.18 |
---|---|
[안드로이드/Android] 안드로이드 중단 오류(카톡/카카오톡, 인터넷, 앱) (0) | 2021.03.23 |
[안드로이드/Android] Error launching studio 오류 해결 (0) | 2021.03.18 |
[안드로이드/Android] XAMPP로 개인 웹 서버 구축해 안드로이드-MySQL 연동하기(3) Apache+MariaDB+PHP (5) | 2021.02.24 |
[안드로이드/Android] XAMPP로 개인 웹 서버 구축해 안드로이드-MySQL 연동하기(2) Apache+MariaDB+PHP (2) | 2021.02.24 |
클라우드, 개발, 자격증, 취업 정보 등 IT 정보 공간
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!